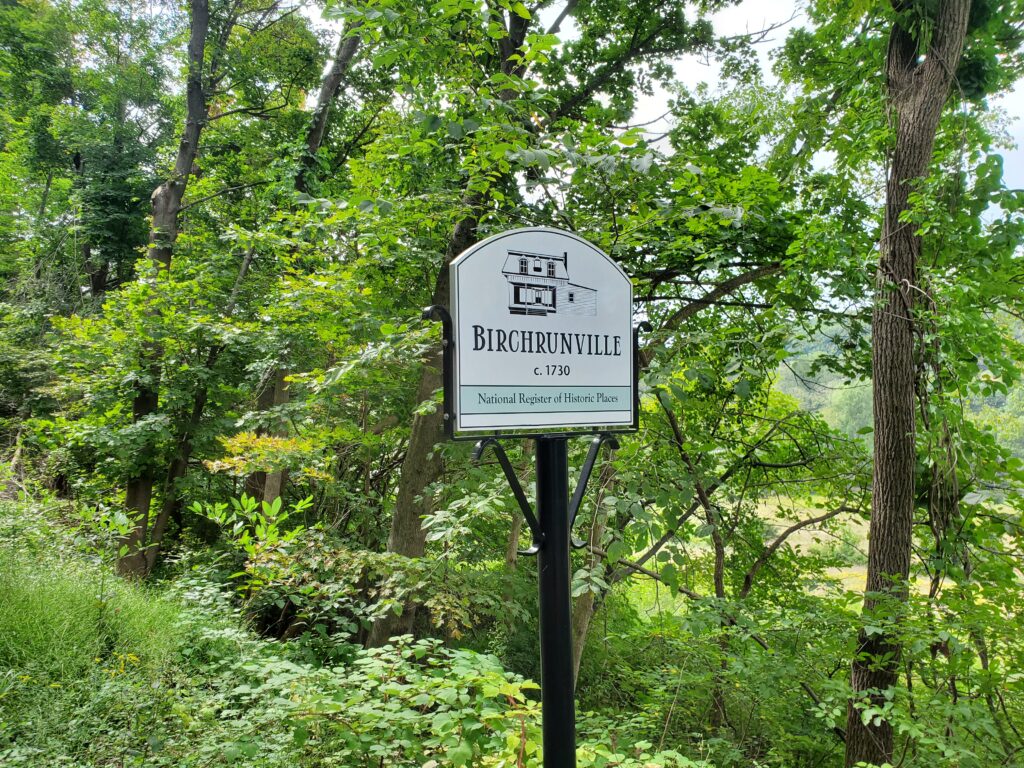
3.74km in 26:30, 7:05/km pace
POSSE: Publish (on your) Own Site, Syndicate Elsewhere
I might start doing this.
It’s been nearly a year since I blogged here, but I’ve made a few posts to the Resources.co blog:
I’m cofounding a new startup, called Resources.co.
It will be a new platform for interacting with data and APIs.
Currently it has a visual JSON editor. If you go to Examples > Pokemon on desktop or paste request get https://pokeapi.co/api/v2/pokemon/bulbasaur
into it on mobile, you can see the JSON editor. It will soon be able to make any kind of API request, like Postman.
I’ll post more about it later!
If you have a homebrew-installed library that you need to link against, use brew –prefix to set your LDFLAGS and CPPFLAGS:
brew install openssl
export LDFLAGS="$LDFLAGS -I$(brew --prefix openssl)/include -L$(brew --prefix openssl)/lib"
export CPPFLAGS="$CPPFLAGS -I$(brew --prefix openssl)/include -L$(brew --prefix openssl)/lib"
I needed this to install the latest version of psycopg2. Before I set this, I was getting this error:
Library not loaded: libssl.dylib
I got part of the way there with this StackOverflow answer comment and the rest of the way with a StackOverflow answer that said to use LDFLAGS (setting DYDL_LIBRARY_PATH didn’t work for me) and the rest of the way from another StackOverflow answer that said to use LDFLAGS.
Sometimes when starting a new project it’s useful to set up a temporary port on vagrant, so a new port can be accessed without running vagrant reload. vagrant ssh can be used for port forwarding. To forward a port, run:
vagrant ssh -- -L 3001:localhost:3000
This will forward port 3001 on the host to port 3000 on the guest.
In esnext, you can use array destructuring to capture the first elements of an array, as well as the rest:
const [a, b, ...c] = [1, 2, 3, 4, 5] console.log([a, b, c]) // [1, 2, [3, 4, 5]]
You can’t, however, put the rest operator at the start or in the middle of the destructuring expression. It must be at the end.
This is a bummer. CoffeeScript supports having a rest argument in the middle of a destructuring expression. Since esnext supports nested array destructuring, this limitation can at least be worked around concisely.
To implement getting the first two, the last two, and the middle, I can define a function leftrest
which takes an array [l1, l2, l3..., r1, r2]
and a number of rightmost arguments to expect, and returns an array with the first element containing the left arguments, followed by the rightmost arguments ([[l1, l2, l3...], r1, r2]
):
const leftrest = (a, n) => { const rest = [...a] return [rest, ...rest.splice(-n)] } const [[a, b, ...c], d, e] = leftrest([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 2) console.log([a, b, c, d, e]) // [ 1, 2, [ 3, 4, 5, 6, 7, 8 ], 9, 10 ]
The first line of the function const rest = [...a]
simply copies the array before it’s handed to splice. Splice modifies an array in place, so it should be copied first. It’s best to use pure functions to avoid unintended side effects.
This isn’t DRY because it’s necessary to pass the number 2
to leftrest, but it’s close.
There aren’t many language translation resources in open source, creative commons, or public domain. I was thinking about how I might start one and remembered that the English Wiktionary has many Spanish words. However, they are only linked in one direction, from the Spanish word to the English word.
How to find the Spanish word? One way is Spanish Wikipedia. For instance on the Instrumentos musicales por clasificación page, there are these words, which are on the English Wiktionary with the corresponding English words:
It seems like this could be used to bootstrap an open source translation dictionary.
The docs for AWS Signature Version 4 are spread across multiple pages. I’m considering writing a library for it, so I’m making a summary here.